Ever wondered why web development feels like juggling flaming chainsaws while riding a unicycle? 🎪 Meet React - the JavaScript framework that turns that circus act into a smooth magic show!
Whether you're building a personal blog or the next big thing in tech, React's got your back with its magical powers of simplification and reusability. Let's dive in and discover why developers worldwide can't stop raving about it!
What is React?
React is like a JavaScript developer's essential companion. Created by the team at Meta (formerly Facebook), it's a powerful tool that takes the headache out of building modern web apps. Think of it as your go-to toolkit that makes creating beautiful, interactive user interfaces feel like a breeze.
Now, you might be wondering - what's the big deal?
Well, imagine you're building with LEGO blocks. A library like React is like getting a box of pre-made LEGO pieces that snap together perfectly.
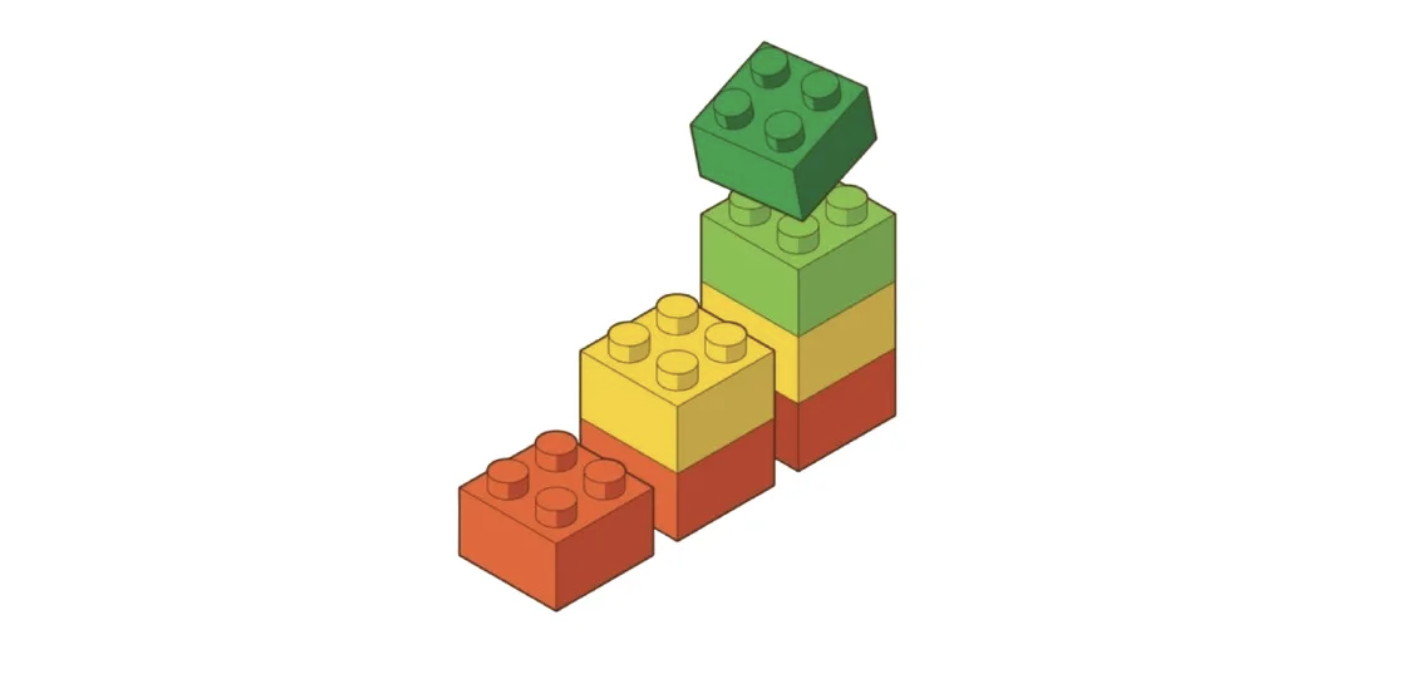
Instead of crafting every tiny piece from scratch, you get these ready-to-use components that save you tons of time and headaches.
But here's where React really shines - it's not just for websites! You can use it to build mostly anything with a user interface.
Want to create a mobile app?
React has a mobile-friendly cousin, React Native. It's like having a universal remote that works on different devices - write your code once, and it works everywhere!
The truly magical thing about React is that we don't have to worry about keeping our state (held in JavaScript) and the DOM* in sync.
With React, we're describing what we want the UI to be, based on the current application state. React takes those descriptions and makes it real.
Hello React
We start with two files: an index.html
file containing a basic HTML document, and an index.js
file with a minimal React application.
Running this code displays a simple paragraph with the text "Hello World!".
1. Import dependencies
import React from 'react';
import { createRoot } from 'react-dom/client';
Let's look at what's happening at the top of our file. We've got two import statements that bring in the tools we need. First, we get React itself (consider it to be the brain of our app), and then we grab something called createRoot
from react-dom (think of this as the messenger between React and your web browser).
Now, here's something cool - React is like a master chef who can cook in any kitchen. It's not tied to just websites! We call this being "platform-agnostic". You've got:
react-dom
for building websitesreact-native
for creating mobile apps (both iPhone and Android) and desktop appsreact-three-fiber
for making remarkable 3D graphics
Each platform has its building blocks (we call them "primitives"). When you're building a website, you use familiar HTML stuff like <div>
, <p>
, and <button>
. But if you're making a mobile app with React Native, you'll use different blocks like Text
and View
. And if you're creating 3D stuff? You get to play with cool things like lights, shapes, and cameras!
The beauty is that React's core stays the same - it's like speaking the same language everywhere. You just need the right translator (we call it bindings) for each platform.
And here's the best part: once you learn React, you can build almost anything! Want to make websites today and mobile apps tomorrow? No problem - your React skills have got you covered!
The DOM (Document Object Model) is the dynamic structure that powers live websites and web applications. When you visit a website, your browser converts the static HTML code into this interactive DOM. When we use a tool like React, it works by interacting with the DOM via JavaScript. It'll create, update, and delete DOM elements as required.
2. Create a React element
Next up in our mini-application, we have the following code:
const element = React.createElement(
'p',
{ id: 'hello' },
'Hello World!'
);
React.createElement
is a function that takes three main arguments:
- The element type you want to create
- Any properties (props) you aim to give the element
- The element's content (which can be left empty)
When this function runs, it creates what we call a "React element" — just a regular JavaScript object. Want to see what it looks like?
Try running console.log(element)
to peek under the hood:
{
type: "p",
key: null,
ref: null,
props: {
id: 'hello',
children: 'Hello World!',
},
_owner: null,
_store: { validated: false }
}
Think of this JavaScript object as a blueprint for what we want to build. It's like telling React "Hey, I want a paragraph with an ID of hello
that says "Hello World!"
". React then takes this blueprint and turns it into the actual paragraph you'll see in your browser.
Pretty neat, right? 🔥
3. Render the application
We've made it to the last few lines of code:
const container = document.querySelector('#root');
const root = createRoot(container);
root.render(element);
document.querySelector
is a versatile function that finds and retrieves existing DOM elements. If you're familiar with JavaScript, you might know its older cousin document.getElementById
.
This function works here because our index.html
file contains the target element:
<div id="root"></div>
This element will be our application's container. When we render our React application, it will create and append new DOM element(s) to this container.
Here's where the magic happens! We use createRoot
from react-dom
to set up our app's foundation - think of it as planting the seed of your React app. Then, with a simple root.render(element)
, we bring our creation to life!
Want to know what's happening behind the scenes? The render
function is like a magical translator - it takes our React blueprint (that JavaScript object we created) and transforms it into something your browser can understand and display. In our case, it's taking our simple paragraph tag, complete with its ID and text, and turning it into real, visible content on your webpage:
<p id="hello">
Hello World!
</p>
Building your own react
One of the best ways to learn how something works is to build our own tiny version of it.
In this exercise, you'll create a
render
function that takes React elements and produces the equivalent DOM structure!For simplicity, we'll skip the
createRoot
API we just discussed. Instead, we'll create a basic render
function that takes two parameters:1. The React element, describing the UI we want.
2. A target DOM node that will house our application.
This is meant to be a challenging exercise 🥵. It's 100% OK if you can't solve it. Spend 5 minutes on it, and then look at the solution code.
Acceptance criteria:
→ The “Result” pane should show the UI described by the React element. It should contain an anchor tag, which links to Yahoo, and contains the text “Visit yahoo website”.
→ It should work with any element type (eg. anchors, paragraphs, buttons…)
→ It should handle all HTML attributes (eg.
href
, id
, disabled
…)→ The element should contain the text specified under
children
. children
will always be a string.Code Playground
Use this playground to code your solution for the practice exercise
Solution code
Here is the solution for the exercise above:
function render(reactElement, containerDOMElement) {
// 1. Create a DOM element
const domElement = document.createElement(reactElement.type);
// 2. Update properties
domElement.innerText = reactElement.children;
for (const key in reactElement.props) {
const value = reactElement.props[key];
domElement.setAttribute(key, value);
}
// 3. Put it in a container
containerDOMElement.appendChild(domElement);
}
const reactElement = {
type: 'a',
props: {
id: 'link',
'data-number': 30,
href: '<https://yahoo.com/>'
},
children: 'Visit yahoo website',
};
const containerDOMElement = document.querySelector('#root');
render(reactElement, containerDOMElement);
Final Words
In this guide to React, we've explored the core concepts that make this JavaScript library a powerful tool for modern web development. We've covered everything from React elements and DOM manipulation to building a simplified version of React's render function, providing essential knowledge for developers at all levels.
Understanding React's platform-agnostic nature, efficient DOM manipulation, and component creation process equips you with the fundamental skills needed to build modern, efficient web applications. The practical exercise reinforces these concepts, helping you become a more capable React developer.
🔍. Similar posts
How to Use Custom Hooks in React
01 Jun 2025
Understanding Stale Values in React
17 May 2025
Write Cleaner and Faster Unit Tests with AssertJ as a Java Developer
12 May 2025