This article provides an overview of the architecture of Spring Boot, a module developed on top of the core Spring Framework that makes it easy to create stand-alone, production-grade Spring-based applications.
The document explains the layered architecture of Spring Boot and the specific functions of each layer, including the controller layer
, service layer
, repository layer
, and database layer
.
I also discuss the advantages and disadvantages of this architecture and offer my brief opinion on that architecture.
What is Spring Boot
Spring is a module developed on top of the core Spring Framework. It makes it easy to create stand-alone, production grade Spring-based Applications that can “just run”. It is also designed to remove XML and annoted-based configuration from the application.
Spring Boot Architecture
Spring Boot follows a layered architecture in which each layer communicates to other layers (above and below in hierarchical order). The spring boot official documentation, provides this code structure for his architecture.
com
+- example
+- myapplication
+- MyApplication.java
|
+- customer
| +- Customer.java
| +- CustomerController.java
| +- CustomerService.java
| +- CustomerRepository.java
|
+- order
+- Order.java
+- OrderController.java
+- OrderService.java
+- OrderRepository.java
But in many enterprise applications, this structure has evolved to something like that ↓
com
+- example
+- myapplication
+- MyApplication.java
+- Entity
| +- Customer.java
| +- Order.java
+- Repository
| +- CustomerRepository.java
| +- OrderRepository.java
+- Service
| +- CustomerService.java
| +- OrderService.java
+- Controller
| +- CustomerController.java
| +- OrderController.java
+- resources
+- application.yml
This is just the basic structure of a spring boot application, but, they are other packages that can be added to this folder architecture like Model, Utils, Enum, Config, etc…
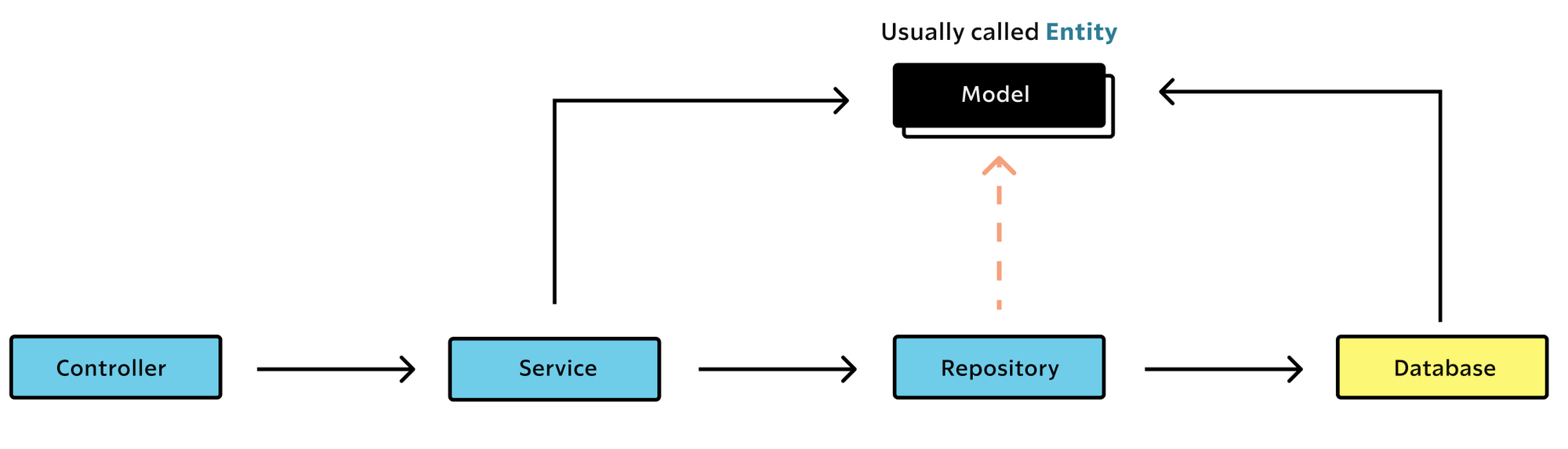
We will not be focusing on that in this article, but only on the basic structure. So let’s see what are the specificities of every layer.
Controller
The controller layer handles the HTTP Requests, translates JSON parameters to object, also defines endpoints for the API. Endpoints can be seen as valid routes and request methods — GET , POST, PUT , PATCH, DELETE.  The controller's main goal is to provide response or offer services to the client (frontend).
Service
The Service layer implement business logics. It consists of service classes and works on data provided by data access layers. It also performs authorization and validation. All sort of computations on the data are performed in this layer. Thus, this layer relies on the Repository Layer.
Repository
Also known as the Persistence / DAO Layer, the main goal of this layer is to access (Query) data from the database and provide them to the service layer. This layer contains all the storage logic and translates business objects from and to database rows.
Database
This layer contains all the databases such as MySQL, PostgreSQL, MongoDB, H2, etc. This is also where the data is stored and retrieved by the repository layer.
My Hot Take on This Architecture
Honestly, I think it’s a good architecture easily understandable by beginners, and it can do the job for of the apps we want to build.
But in the software craftsman sphere, (and in my opinion) the Hexagonal Architecture seams more appropriate for enterprise applications.
Another thing, I don't necessarily agree with the fact that clean architecture gurus denigrate a developer's skills if they don't fit into their sphere of  "craftsmanship". Of course, it’s good to know some advanced concepts in your field, but in my opinion it’s not mandatory to do a good job.
Even though this architecture is easy to understand and to use, It has some cons, it can be hard to build large-scale apps because it is unsuitable for working with microservices for example.
Ending
Spring Boot follows a layered architecture (Repository Pattern) that allows for clear separation of concerns and easier maintenance and development.
- The controller layer handles HTTP requests and defines endpoints for the API
- The service layer implements business logic and performs computations on the data provided by the repository layer.
- The repository layer accesses data from the database layer, which contains all the databases and stores and retrieves data.
Spring Boot's architecture is designed to be easy to use and understand, and its modular structure allows for flexibility and customization. Overall, understanding Spring Boot's architecture is crucial for any developer looking to build robust and scalable applications.
🔍. Similar posts
Understanding the useRef hook in React
28 Apr 2025
React Component Cleanup
12 Mar 2025
How to Run Code on Mount in Your React Components Easily
04 Mar 2025