Managing state in React can be like navigating a maze, particularly as your application becomes more complex. When state is scattered across components, keeping everything synchronized is challenging.
This scattered approach makes your codebase harder to maintain and more prone to bugs, which can undermine your application's reliability. These challenges often distract from what matters most — building great new features.
Thankfully, there's a solution: ✨ Lifting State Up ✨ ** technique provides a clear approach to state management by centralizing your application's data and ensuring all components receive consistent information. With this strategy, you can transform scattered state management into streamlined, efficient React development.
How to lift the React state
Let consider the playground below, we want to render the result of the search box in the component below him. But we cannot do that because the state data searchTerm
lives in the SearchForm.js
component.
Let’s consider this diagram, that shows a parent relationship between 3 components. First we will have App.js
, which renders 2 children component, it renders SearchForm.js
and SearchResult.js
.
SearchForm.js
is the one that holds the state for the actual searchTerm
.
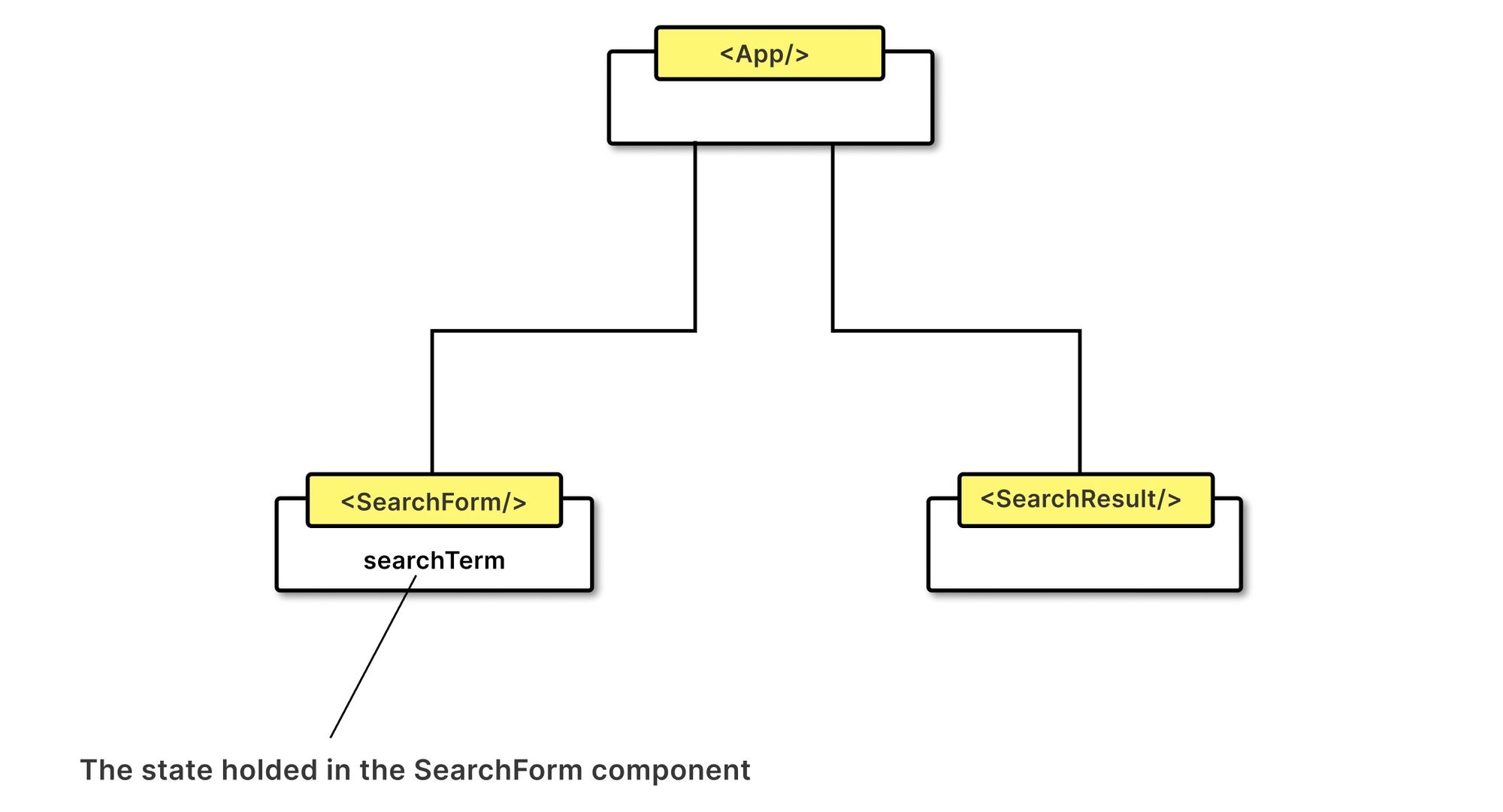
Now we want to access the data searchTerm
from the SearchForm.js
in the SearchResult.js
components. React solves this problem elegantly, which is the rule in React is that data can only pass through components via props or via context.
In this blog post, we will focus on passing data via context.
To solve this problem, we need to lift the state up. This means moving the state from the SearchForm.js
component higher up in the component tree. Once the state is lifted, the parent component can pass it down to any child component that needs it—this is how React's data flow works.
They also call this Unidirectional Data Flow. This basically means parents can pass data to children, but children cannot pass data to parents, and siblings can’t pass data to siblings. It's always going in this singular direction.
So to lift the state, we have to move the searchTerm
state from the SearchForm.js
child component to the parent component App and pass that state as a prop in the children components SearchForm.js
and SearchResult.js
.
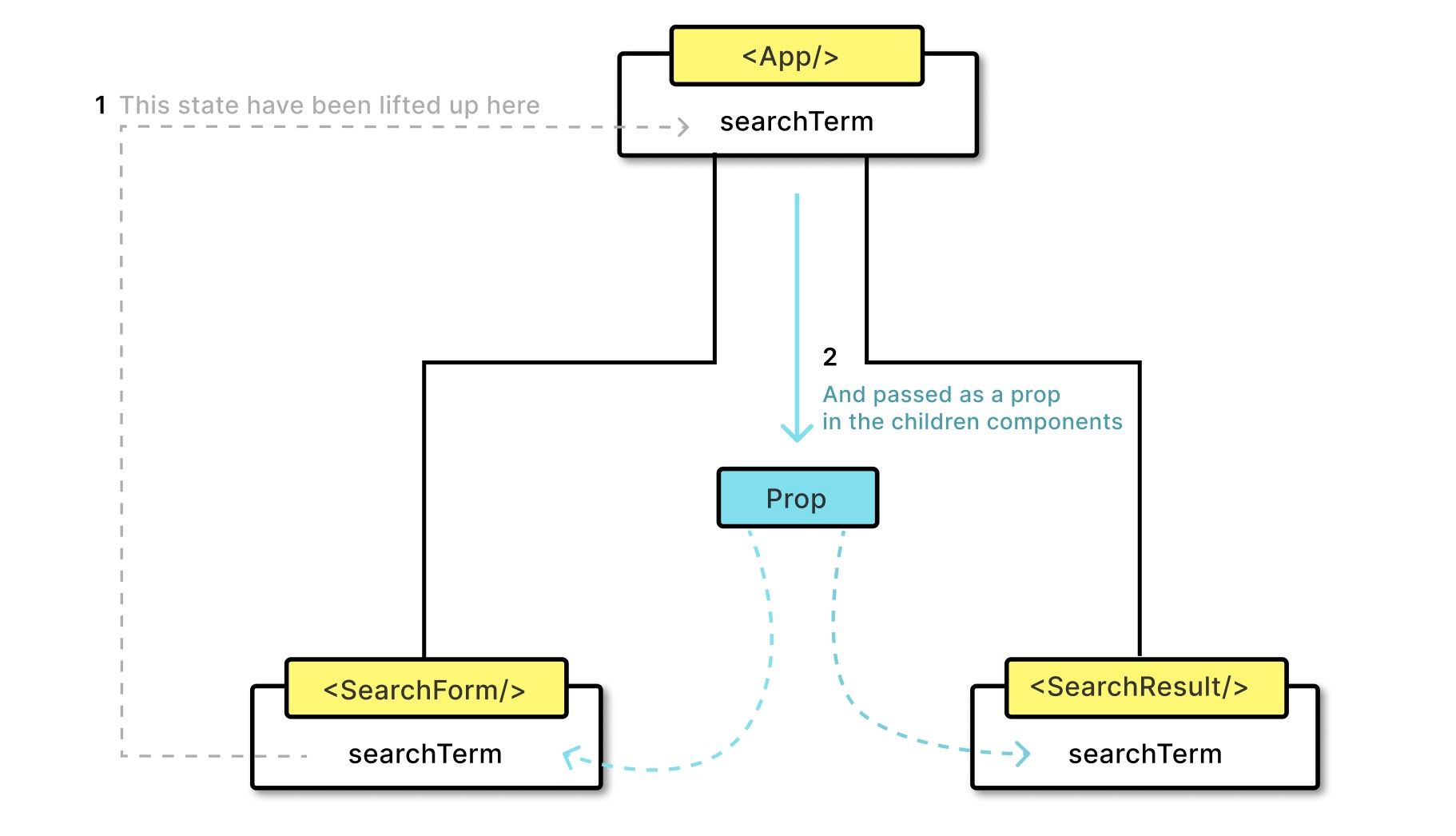
React get a bit clever here! It plays with the idea that functions in JavaScript are data just like any other, that’s one of the things that make JavaScript a functional programming language.
So in our case, we have to pass the function
setSearchTerm
as a prop in the SearchForm.js
children and use it to update the state in the parent component.In the demo sandbox below you can see how the state data from the SearchForm.js
component is lifted into the App component.
Lifting state hierarchy
The best practice when we want to lift the state up, is to lift it up to the closest shared ancestor. In other words, we only want to re-render the components that require the data, on the whole application.
Summary
In React, state is primarily passed through props, flowing from parent components to their children. Sibling components cannot share state directly with each other. To share state between siblings, we lift it up to their common parent component, which can then distribute it to both children.
Along with the state, we can pass state-setter functions down to children, enabling them to update the parent's state.
Here's how this process works step by step:
- A user types in the text box, triggering a change event.
- The state setter function (received as a prop) is called.
- Since the state belongs to the
App
component, it triggers a re-render, updating bothSearchForm
andSearchResults
SearchForm
receives and displays the updatedsearchTerm
valueSearchResults
receives and displays the same updatedsearchTerm
value
Why not lift all state to the very top of the application? This would be impractical for anything but the smallest apps, causing performance issues and unnecessary complexity.
🔍. Similar posts
How to Effectively Use the React useId Hook in Your Apps
16 Feb 2025
How to Master Dynamic Key Generation in React for Your Apps
12 Feb 2025
How to Fix Mutation Bugs in Your React Complex State Logic
10 Feb 2025