In the ever-evolving landscape of web development, containerization has become a crucial aspect of deploying applications seamlessly across different environments.
Docker, a leading containerization platform, offers a robust solution for packaging and distributing applications.
This guide explores how to run a Node.js application in a Docker container while efficiently managing environment variables using a dedicated file.
Create an Environment File
Start by creating an environment file, typically named .env
. This file will store key-value pairs for your application's environment variables. For instance:
NODE_ENV=production
PORT=3000
Customize these variables according to your specific requirements.
Generate Source Files
Open your terminal and navigate to the project folder, run this command to generate a basic express node project.
npx express-generator
This command will generate all the sources file you need to start and run a basic nodejs app.
Dockerfile Configuration
Create a Dockerfile in the root of your project to provide instructions for building the Docker image. Name that file node.dockerfile
and add the following code :
# Use an official Node.js runtime as a parent image
FROM node:20-alpine
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy package.json and package-lock.json to the working directory
COPY package*.json ./
# Install app dependencies
RUN npm install
# Bundle app source
COPY . .
# Show the port the app runs on
EXPOSE 3000
# Command to run the application
CMD ["npm", "run", "start"]
Adjust the Node.js version and other settings as per your project's requirements.
Build the Docker Image
Open a terminal, navigate to your project's directory, and run the following command to build the Docker image:
docker build -f node.dockerfile -t node-app . --no-cache
Replace node-app
with a suitable name for your Docker image.
Run the Docker Container
Now, use the docker run
command to start the container, including the environment file:
docker run --env-file .env -p 3000:3000 node-app -d
This command sets the environment variables from the .env
file and maps port 3000
from the container to port 3000 on your host machine.
Now go on your favorite browser and launch this URL localhost:3000
. You will have this page showing up, telling you that your nodejs app is up and running.
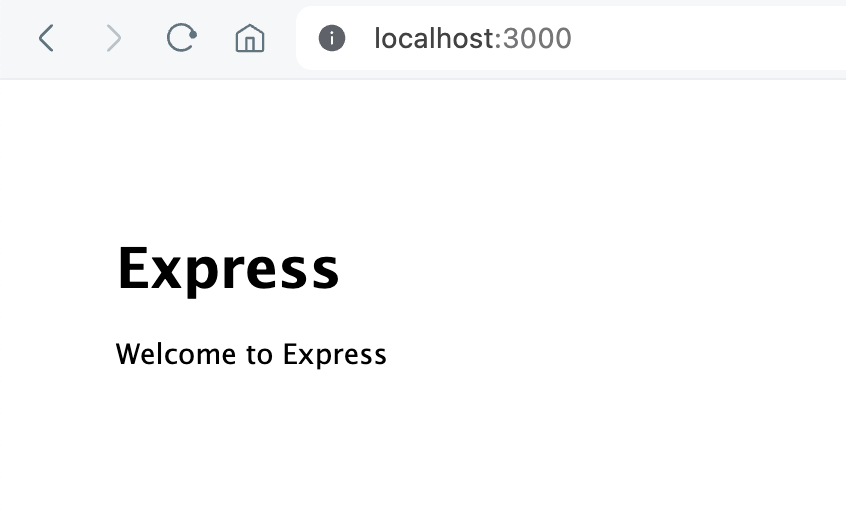
Ending
In conclusion, containerizing Node.js applications with Docker provides a scalable and portable solution for deployment. By utilizing environment variables stored in a dedicated file, you can easily manage configuration settings across different environments.
This tutorial has provided a practical guide on how to launch a Node.js application in a docker container. You can all the source code on my GitHub.
🔍. Similar posts
How to Use Custom Hooks in React
01 Jun 2025
Understanding Stale Values in React
17 May 2025
Write Cleaner and Faster Unit Tests with AssertJ as a Java Developer
12 May 2025