Calling functions within functions, or violating software design rules, are very common when you're just starting out.
You've probably already been in the situation where you've written procedural code without necessarily declaring variables to store your data.
Yet, variables are essential components of any programming language, including JavaScript. They are used to storing and manipulating data that is used throughout an application.
In this article, we will discuss the different types of variables in JavaScript and how to declare and use them in your code. We will also cover important concepts such as block and global scopes.
Variables Declarations
We declare variables to store data by using the keywords var
, let
, const
keywords.
let
– is a modern variable declaration.var
– is an old-school variable declaration.const
– is likelet
, but the value of the variable can’t be changed.
Keyword let
To create (declare) a variable in JavaScript, you can use the let
keyword.
let information;
Now, we can put some data into it by using the assignment operator =
:
let information = "Hello you";
You can declare multiple variables, by declaring each of them in a single line:
let user = 'John';
let age = 25;
let message = 'Hello motherfucker';
Declaring a variable twice triggers an error → a variable should be declared only once, a repeated declaration of the same variable is an error.
let information = 'coco banjo';
// repeated 'let' leads to an error
let information = 'baba banjo'; // SyntaxError: 'message' has already been declared
Keyword const
To declare a constant (unchanging) variable, use const
instead of let
:
const myAddress = 'Sherbrooke, Canada';
Variables declared using const
are called constants. They cannot be reassigned. An attempt to do so would cause an error:
const myBirthday = 'Sherbrooke, Canada';
myBirthday = 'Frankfurt, Germany'; // error, can't reassign the constant!
When a programmer is sure that a variable will never change, they can declare it with const
to guarantee and clearly communicate that fact to everyone.
There is a widespread practice to use constants as aliases for difficult-to-remember values that are known before execution.
Such constants are named using capital letters and underscores.
For instance, let’s make constants for colors in so-called “web” (hexadecimal) format:
const COLOR_BLUE = "#00F";
const COLOR_ORANGE = "#FF7F00";
// ...when we need to pick a color
let color = COLOR_ORANGE;
console.log(color); // #FF7F00
Benefits:
COLOR_ORANGE
is much easier to remember than"#FF7F00"
.- It is much easier to mistype
"#FF7F00"
thanCOLOR_ORANGE
. - When reading the code,
COLOR_ORANGE
is much more meaningful than#FF7F00
.
Let’s make that clear.
Being a constant just means that a variable’s value never changes. But there are constants that are known before code execution (like a hexadecimal value for red) and there are constants that are calculated at run-time, during the execution, but do not change after their initial assignment.
In other words, capital-named constants are only used as aliases for
hard-coded
values.For instance:
const pageLoadTime = /* time taken by a webpage to load */;
The value of pageLoadTime
is not known before the page load, so it’s named normally. But it’s still a constant because it doesn’t change after assignment.
Variables Naming Rules
There are two limitations on variable names in JavaScript:
- The name must contain only letters, digits, or the symbols
$
and_
. - The first character must not be a digit.
Variables should be named in a way that allows us to easily understand what’s inside them. Variable naming is one of the most important and complex skills in programming.
A quick glance at variable names can reveal which code was written by a beginner versus an experienced developer. Please spend time thinking about the right name for a variable before declaring it. Doing so will repay you handsomely 😉.
When the name contains multiple words, camelCase is the recommended syntax to use. example → randomLongName.
What’s interesting – the dollar sign $
and the underscore _
can also be used in names. They are regular symbols, just like letters, without any special meaning.
These names are valid:
let $ = 10; // declared a variable with the name "$"
let _ = 25; // and now a variable with the name "_"
console.log($ + _); // 35
Examples of incorrect variable names:
let 1a; // cannot start with a digit
let my-name; // hyphens '-' aren't allowed in the variable name
There is a list of reserved words, which cannot be used as variable names because they are used by the language itself. For example: let, class, return, and function are reserved.
The code below gives a syntax error:
let let = 50; // can't name a variable "let", error!
let return = 25; // also can't name it "return", error!
Variables Scopes
Scope determines the accessibility (visibility) of variables. JavaScript has 3 types of scope:
- Block scope
- Function scope
- Global scope
Block scope
The block scope of a variable means that the variable is accessible within the block that is between the curly braces.
Consider the code below:
if (true) {
let y = 15;
var x = 40;
console.log(x);
console.log(y);
}
console.log(x);
console.log(y);
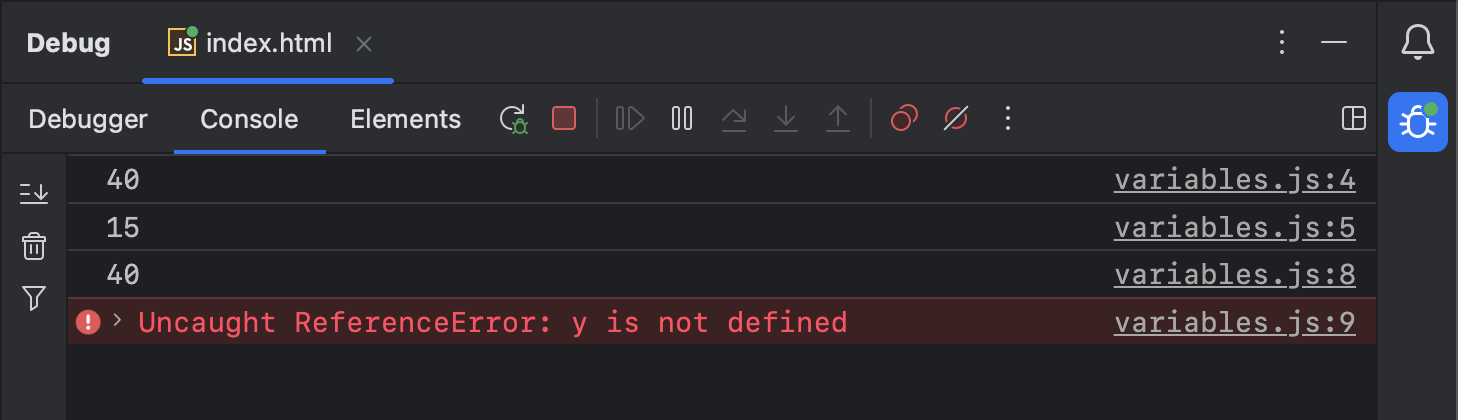
Variables declared with the
var
keyword can NOT have block scope. These variables declared inside a {}
block can be accessed from outside the block.Function scope
Variable declared within a JavaScript function, becomes Local
to the function. Each function creates a new scope. Variables defined inside a function are not accessible
// code here can NOT use barName
function randomFunction() {
let barName = "Irish Pub";
// code here CAN use barName
}
// code here can NOT use barName
Since local variables are only recognized inside their functions, variables with the same name can be used in different functions. Local variables are created when a function starts, and deleted when the function is completed.
Global scope
A variable declared outside a function, becomes Global
. They can be accessed from anywhere in a JavaScript program. Variables declared with var
, let
and const
are quite similar when declared outside a block.
let carName = "Tesla";
// code here can use carName
function carFunction() {
// code here can also use carName
}
The life of a JavaScript variable starts when it is declared. Function (local) variables are deleted when the function is completed. In a web browser, global variables are deleted when you close the browser window (or tab).
Function arguments (parameters) work as local variables inside functions.
Wrap Up
In this article, we have learned about the different types of variables in JavaScript and how to declare and use them in your code. Remember that var
, let
, and const
are used to declare variables.
var has a function scope, while let and const have block scope. const
is used to declare a constant variable, while let
is used for regular variables.
🔍. Similar posts
Why Are My React Components Re-rendering Too Much?
26 Jul 2025
How to Use Custom Hooks in React
01 Jun 2025
Understanding Stale Values in React
17 May 2025